This documentation provides a complete overview for developing your own integrations with Snapforce CRM. We explain the many uses other organizations use our API for, and present quick and easy examples. This documentation also explores the available methods at your disposal and real world code examples you can use to get your integration going. The examples in this documentation are written in the PHP programming language, but have recently published an official Perl module, Snapforce::API, on CPAN for easy integration into a Perl application. You can also find us on Stack overflow, answering questions users have about using the api.
Many customers want to utilize the Snapforce API to keep their data in sync with a proprietary application they are running, or to share data with a third-party service they are using, but whatever the reason - there are many useful ways to programmatically integrate your CRM.
Getting Started
To get started, simply create your API access tokens inside Snapforce under the Developers page in your Administrator Setup area. You can create your initial access token and refresh it as often as needed. Once you complete that, the fun part begins – dive into the Snapforce API Documentation over the following pages and begin building your integrations!
Introduction
Snapforce CRM provides a RESTful web API, or "Application Programming Interface", so customers can programmatically control all aspects of their data; inserts, updates, deletes, syncing etc. The only time you will need to use this API is if you are integrating another system, whether it be your company's proprietary systems, your erp, accounting software etc. Less commonly, users may wish to manipulate their CRM's data from an external application, like an Ipad or their users mobile devices. Additionally, the Snapforce API architecture always allows for you to make calls to your CRM, there is no limit on the amount of usage the API can be utilized for. The Snapforce culture believes that our customers should be able to access their data easily and however they wish, we do not believe in charging our users to access their data or make it difficult to do so.
The API in total contains over a half dozen calls that can be made and allows for filtered logic to be passed via the calls as well, which helps you (the programmer) to receive only the data you want and not have to manually parse and write a large amount of additional code to retreive the data you sought out in the first place to obtain. Most users of the API use only a few of the primary functions when implementing their integrations, and when they use these core functions in certain processes it can allow for a very robust workflow.
The remainder of this documentation walks you through the common workflows and gives PHP implementation examples.
If you are looking to quickly get your feet wet and setup a simple but useful integration we detail three of the most widely used API workflows below. In the three examples you will find code samples, syntax specifics, and explanatory information about these common workflows. You can also find all of the examples on github. If you are programming a much deeper or complex integration than feel free to skip the below (primary workflows) section and move on to the full Snapforce CRM API documentation.
Pull Data From the CRM
The first workflow you may wish to implement is the "fetchRecords()" flow, which is simple and useful if you need to pull data out of Snapforce.
With the "fetchRecords()" flow you can (as an example) pull a list of Accounts, or a list of your newest leads, etc. This is one of the most widely used and basic processes of the API. An example of implementation using the PHP programming language can be found below.
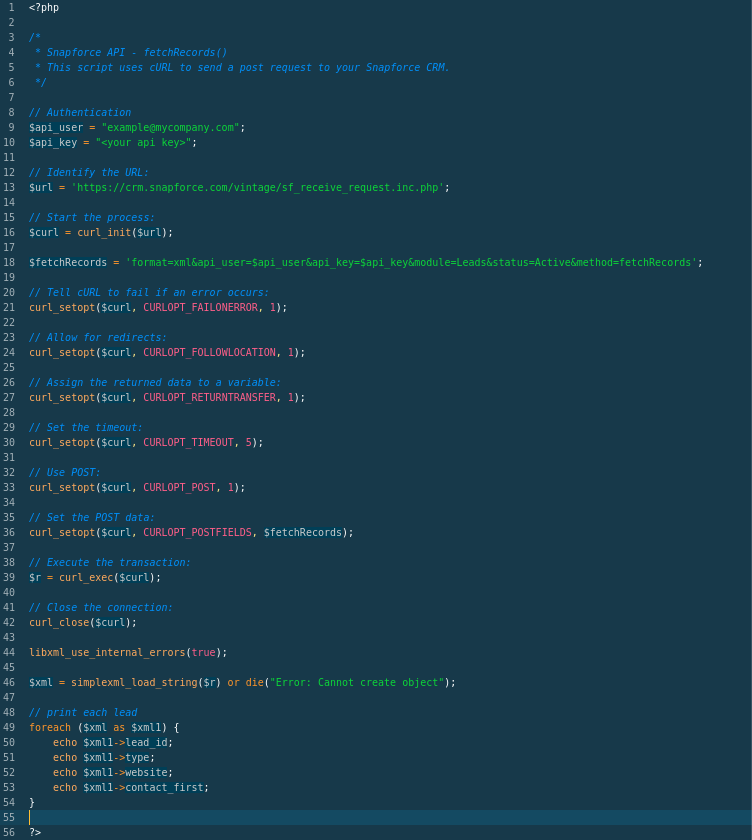
Insert Data to the CRM
The second workflow you may wish to implement is the "insertRecords()" flow, which is simple and useful if you need to push a lot of data into Snapforce.
With the "insertRecords()" flow you can (as an example) pull of the orders for the day out of your accounting system and insert them into your CRM system. This is one of the most widely used and basic processes of the API. See the correct XML structure for sending an insertRecords() request via the API.
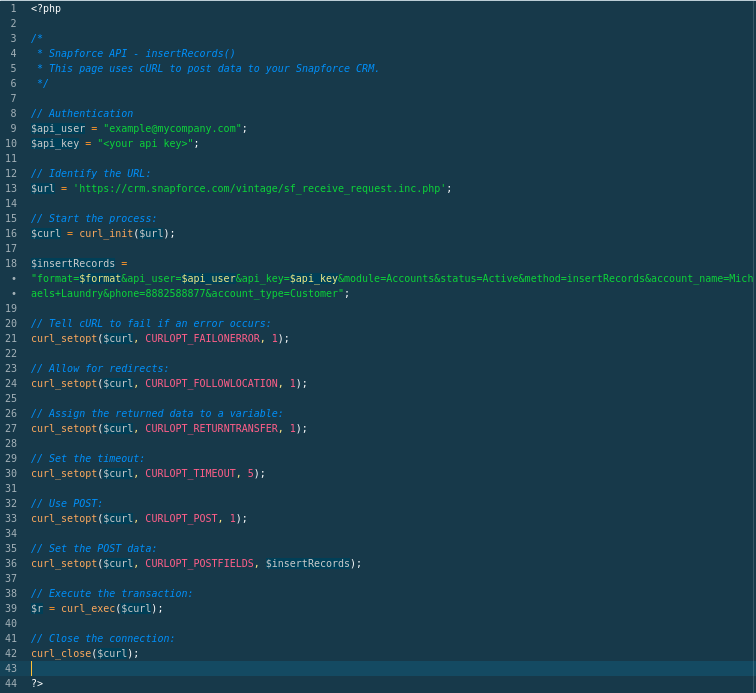
Update Data in the CRM
The third workflow is the "updateRecords()" method, which lets you update existing data in your system.
With the "updateRecords()" method you can update any of the user available standard and custom fields in the available modules. You are able to update a single record or loop through a series of records to update multiple.
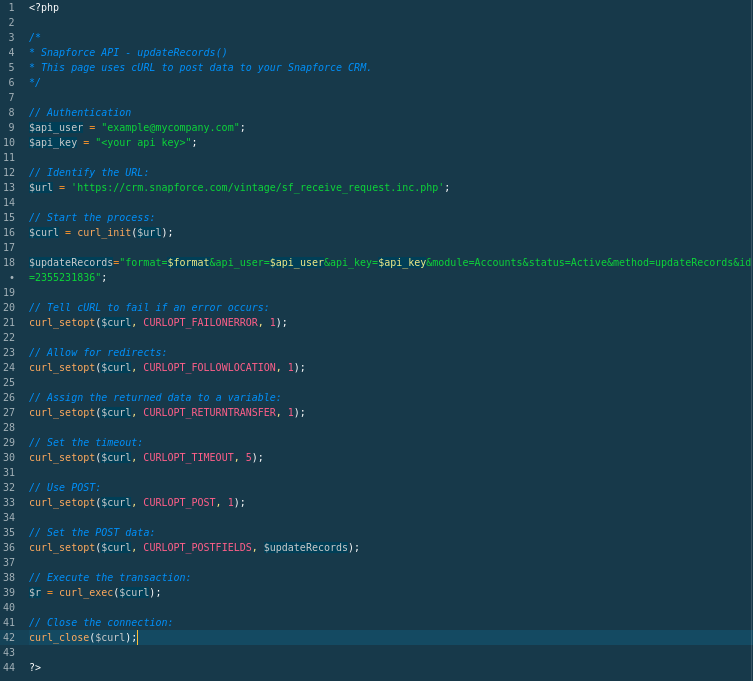